To make a flowing water light, you will need the following:
- 11 wires
- 1 bar graph LED
- 1 breadboard
- 10 220 ohm resistors
- 1 raspberry pi 3
Now, place the pieces like the following:
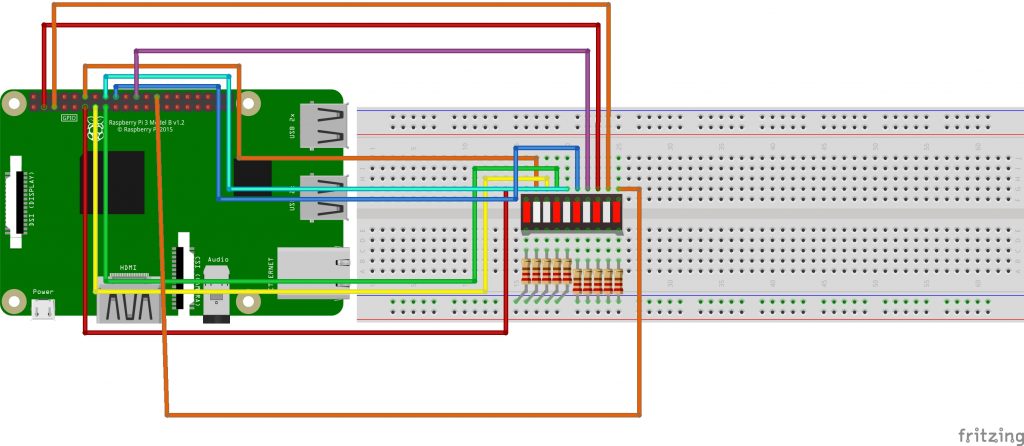
Once you are done putting the pieces in the right place, type the following code:
import RPi.GPIO as GPIO
import time
ledPins = [11, 12, 13, 15, 16, 18, 22, 3, 5, 24]
def setup():
GPIO.setmode(GPIO.BOARD)
GPIO.setup(ledPins, GPIO.OUT)
GPIO.output(ledPins, GPIO.HIGH)
def loop():
while True:
for pin in ledPins:
GPIO.output(pin, GPIO.LOW)
time.sleep(0.1)
GPIO.output(pin, GPIO.LOW)
for pin in ledPins[::-1]:
GPIO.output(pin, GPIO.LOW)
time.sleep(0.1)
GPIO.output(pin, GPIO.HIGH)
def destroy():
GPIO.cleanup()
if __name__ == '__main__':
print('Program is starting... \n')
setup()
try:
loop()
except KeyboardInterrupt:
destroy()
Once you run the program, the LED bar makes a flowing water lamp!
Changing The Program
You can also modify the program so it seems like something that shows the power of something! Just remove line 18 and voilà!