Making a police light from a Raspberry Pi isn’t easy! But it sure is cheap. You will need:
- 7 wires
- 1 raspberry pi 3
- 1 red LED
- 1 blue LED
- 2 220 ohm resistors
- 1 momentary push button
- 1 breadboard
Now place the pieces like the following:
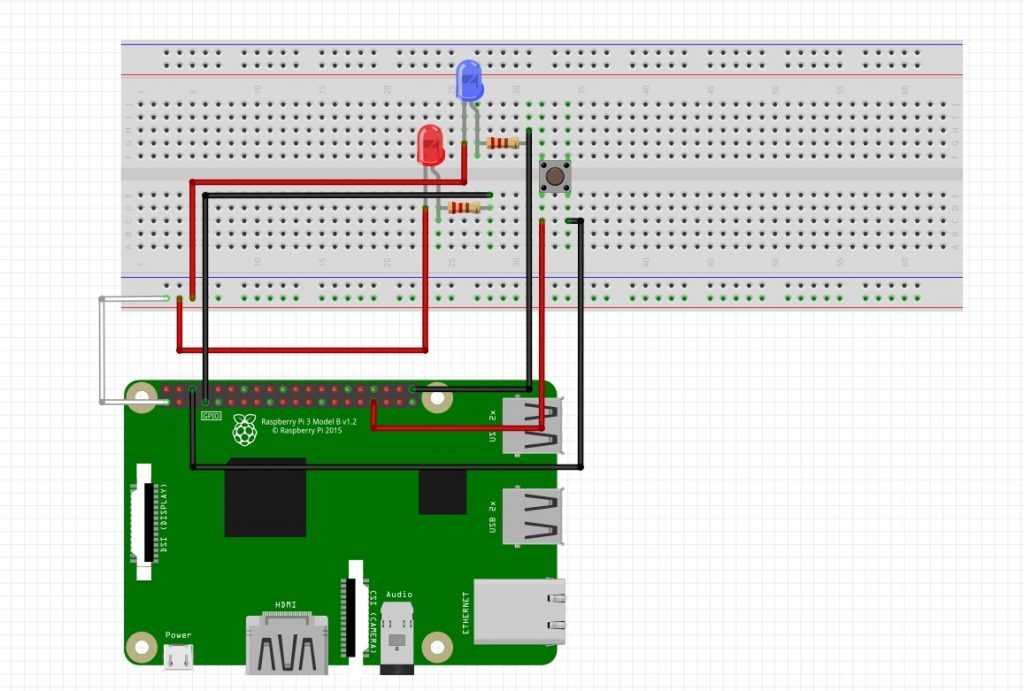
Then, using python, type the following:
# 5/9/2021
"""
This program is connected to a raspberry pi. It works like a police siren. Whenever someone presses the button, it will
go flashing. When the person presses it again, It turns off. It also uses a series of patterns.
"""
import gpiozero # import the needed GPIO library
import time # import this package so the program isn't that fast.
# Get the variables and LEDs ready
button = gpiozero.Button(13)
red_led = gpiozero.LED(4)
blue_led = gpiozero.LED(21)
# Turn the light on or off?
# Boolean variable to represent "On" or "Off"
policeLightSwitch = False
# Turn the LEDs off
# Turns out, the LED on() and off() things do the opposite. Not sure why.
red_led.on()
blue_led.on()
# infinite loop
while True:
# So it doesn't do True, then False, then True...
if button.is_pressed:
if policeLightSwitch:
policeLightSwitch = False
else:
policeLightSwitch = True
if policeLightSwitch: # Light On?
while not button.is_pressed: # terminate the loop when button pressed
for i in range(2): # now for the series of lights!
red_led.off()
time.sleep(0.05)
red_led.on()
time.sleep(0.05)
blue_led.off()
time.sleep(0.05)
blue_led.on()
time.sleep(0.05)
policeLightSwitch = True
policeLightSwitch = False
else:
# Turn the LEDs off
blue_led.on()
red_led.on()
while not button.is_pressed: # terminate when button pressed
policeLightSwitch = False
policeLightSwitch = True
print(policeLightSwitch) # Is the police light supposed to be flashing or not?
If you hadn’t installed gpiozero yet, you will get an error message. You can install it on pycharm by hovering over the “gpiozero” part and click “install package.” Otherwise, when you run it, when you press the button, the LEDs should flash like a police light, and when you press it again, it will stop!