Remote controll
A remote controll uses something that can detect the numbers pressed on a remote controll. It is called the Infrared Receiver. Like in the name, the receiver uses infrared light, which cannot be seen by the naked eye, to detect remote controll signals.

Liquid Crystal Display
An LCD (Liquid Crystal Display) is a type of display. The wiring is nasty, though, and it could take a while.

Wiring
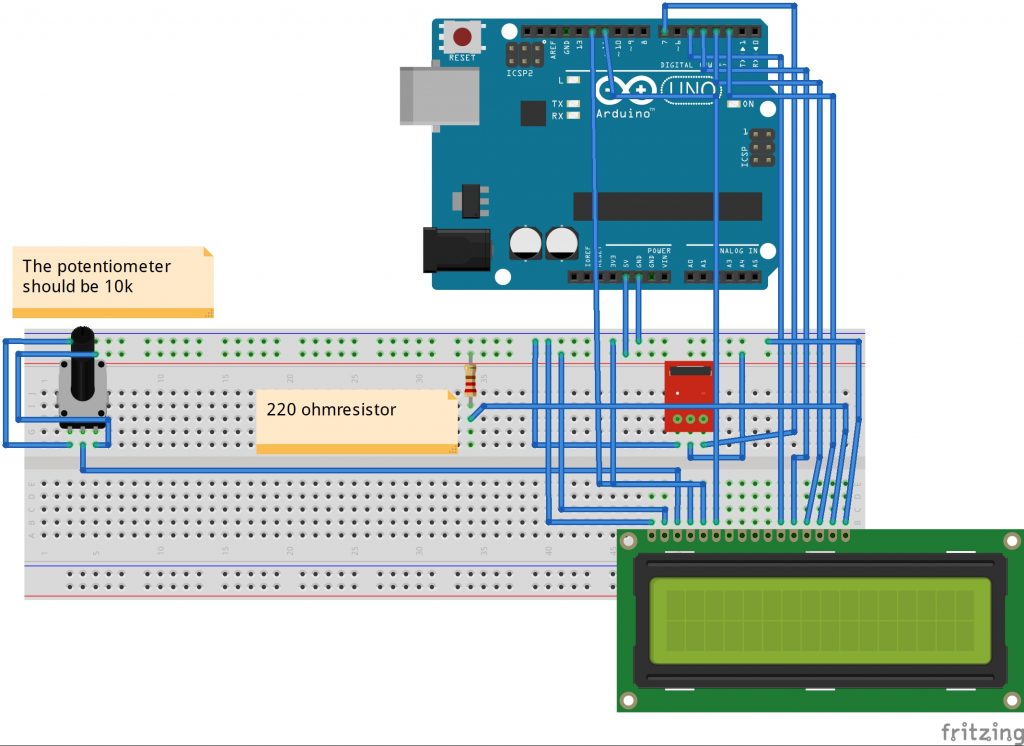
Setting up
You will need to install a package that uses the infrared. Open the Arduino IDE. Go to Sketch > Include Library > Manage Libraries. In the search bar, type in “irremote.” Download the latest version.
Code
First, type in the following code:
#include <IRremote.h>
#include <LiquidCrystal.h>
int IR_RECEIVE_PIN = 7;
const int rs = 12, en = 11, d4 = 5, d5 = 4, d6 = 3, d7 = 2;
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
void setup() {
lcd.begin(16, 2);
lcd.setCursor(0, 0);
Serial.begin(9600);
IrReceiver.begin(IR_RECEIVE_PIN);
lcd.clear();
}
void loop(){
if (IrReceiver.decode())
{
IrReceiver.resume();
int num = IrReceiver.decodedIRData.command;
Serial.println(num);
}
delayMicroseconds(2);
}
When you open up the Serial Monitor and press the number 0, you should see a random number. This is the number you receive when you press 0. Note that the number is different for every device.
After you know all of the numbers for every button, type in a code like this:
#include <IRremote.h>
#include <LiquidCrystal.h>
int IR_RECEIVE_PIN = 7;
const int rs = 12, en = 11, d4 = 5, d5 = 4, d6 = 3, d7 = 2;
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
void setup() {
lcd.begin(16, 2);
lcd.setCursor(0, 0);
Serial.begin(9600);
IrReceiver.begin(IR_RECEIVE_PIN);
lcd.clear();
}
void loop(){
if (IrReceiver.decode())
{
IrReceiver.resume();
int num = IrReceiver.decodedIRData.command;
if (num == 22){
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Hello, World!");
}
else if (num == 12){
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("1+1=2");
}
else if (num == 24){
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("111111*111111=");
lcd.setCursor(0, 1);
lcd.print("12345654321");
}
else if (num == 94){
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Remote");
lcd.setCursor(0, 1);
lcd.print("Controller");
}
else if (num == 8){
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("This is");
lcd.setCursor(0, 1);
lcd.print("# 4!");
}
else if (num == 28){
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Do you know how");
lcd.setCursor(0, 1);
lcd.print("to make this?");
}
else if (num == 90){
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Liquid Crystal");
lcd.setCursor(0, 1);
lcd.print("Display");
}
else if (num == 69){
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Arduino can't");
lcd.setCursor(0,1);
lcd.print("turn off!");
}
else if (num == 70){
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Volume is");
lcd.setCursor(0,1);
lcd.print("going up");
}
else if (num == 64){
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Resuming/");
lcd.setCursor(0,1);
lcd.print("Pausing");
}
else if (num == 21){
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Volume is");
lcd.setCursor(0,1);
lcd.print("going down");
}
else if (num == 25){
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Your IQ is:");
lcd.setCursor(0,1);
lcd.print("INFINITY");
}
else{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("UNRECOGNIZED");
}
}
delayMicroseconds(2);
}